Purpose Of This Tutorial

- 2.2 Every process created on a unix system has a unique PID. 2.3 Elective is a type of variable used in shell scripts. 2.4 Copy command is used to transfer files in an FTP session. 2.5 Unix deals with super user the same way other multiuser system do. 2.6 Runlevel 0 is reserved for the 'shutdown' phase.
- A Unix shell is both a command interpreter and a programming language. As a com-mand interpreter, the shell provides the user interface to the rich set of gnu utilities. The programming language features allow these utilities to be combined. Files containing com-mands can be created, and become commands themselves. These new commands have the.
Loading Ebook: 100 Shell Programs In Unix By Sarika Jain, Shivani Jain. Kupdf.com100-shell-programs-part-ii.pdf - Free download as PDF File (.pdf), Text File (.txt) or read online for free. Scribd is the world's largest social reading and publishing site. Open navigation menu.
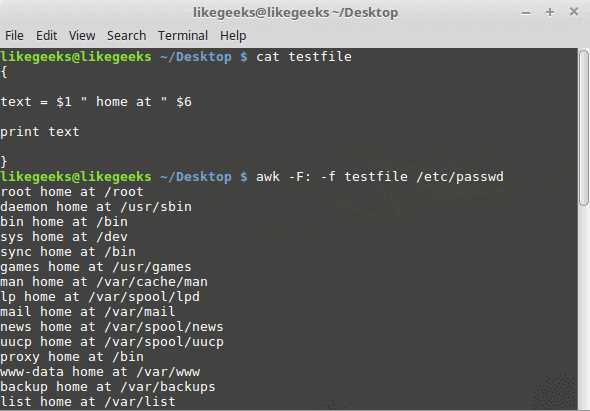
This tutorial is written to help people understand some of the basics of shellscript programming (aka shell scripting), and hopefully to introduce some of the possibilities of simple butpowerful programming available under the Bourne shell. As such, it has been written asa basis for one-on-one or group tutorials and exercises, and as a reference for subsequentuse.
Getting The Most Recent Version Of This Tutorial
You are reading Version 4.2, last updated 2nd March 2021.
The most recent version of this tutorial is always available at:https://www.shellscript.sh.Always check there for the latest copy. (If you are reading this at some different address, it is probably a copy of the real site, and therefore may be out of date).
A Brief History of sh
Steve Bourne wrote the Bourne shell which appeared in the Seventh Edition Bell Labs Research version of Unix.
Many other shells have been written; this particular tutorial concentrateson the Bourne and the Bourne Again shells.
Other shells include the Korn Shell (ksh), the C Shell (csh), and variations such as tcsh.
This tutorial does not cover those shells.
Audience
This tutorial assumes some prior experience; namely:
- Use of an interactive Unix/Linux shell
- Minimal programming knowledge - use of variables, functions, is useful background knowledge
- Understanding of some Unix/Linux commands, and competence in using some of the more common ones. (ls, cp, echo, etc)
- Programmers of ruby, perl, python, C, Pascal, or any programming language (even BASIC) who can maybe read shell scripts,but don't feel they understand exactly how they work.

You may want to review some of the feedback that this tutorial has received to see how useful you might find it.
Typographical Conventions Used in This Tutorial
Significant words will be written in italics when mentioned for thefirst time.
Code segments and script output will be displayed as monospaced text.
Command-line entries will be preceded by the Dollar sign ($). If your prompt is different,enter the command:
Then your interactions shouldmatch the examples given (such as ./my-script.sh
below).
Script output (such as 'Hello World' below) is displayed at the start of the line.
Next: Philosophy
Lab Exercise-4
1) Write a shell script to ask your name, program name and enrollment number and print it on the screen.
Echo “Enter your name:”
Read Name
Echo “Enter your program name:”
Read Prog
Echo “Enter your enrollment number:”
Read Enroll
Clear
Echo “Details you entered”
Echo Name: $Name
Echo Program Name: $Prog
Echo Enrolment Number: $Enroll
2) Write a shell script to find the sum, the average and the product of the four integers entered
Echo “Enter four integers with space between”
Read a b c d
Sum =`expr $a + $b + $c + $d`
Avg =`expr $sum / 4`
Dec =`expr $sum % 4`
Dec =`expr ($dec * 1000 ) / 4`
Product =`expr $a * $b * $c * $d`
Echo Sum = $sum
Echo Average = $avg. $dec
Echo Product = $product
3) Write a shell program to exchange the values of two variables
Echo “Enter value for a:”
Read a
Echo “Enter value for b:”
Read b
Clear
Echo “Values of variables before swapping”
Echo A = $a
Echo B = $b
Echo Values of variables after swapping
a = `expr $a + $b`
b = `expr $a – $b`
a = `expr $a – $b`
Echo A = $a
Echo B = $b
4) Find the lines containing a number in a file
Echo “Enter filename”
Read filename
Grep [0-9] $filename
5) Write a shell script to display the digits which are in odd position in a given 5 digit number
Echo “Enter a 5 digit number”
Read num
n = 1
while [ $n -le 5 ]
do
a = `Echo $num | cut -c $n`
Echo $a
n = `expr $n + 2`
done
6) Write a shell program to reverse the digits of five digit integer
Echo “Enter a 5 digit number”
Read num
n = $num
rev=0
while [ $num -ne 0 ]
do
r = `expr $num % 10`
rev = `expr $rev * 10 + $r`
num = `expr $num / 10`
done
Echo “Reverse of $n is $rev”
7) Write a shell script to find the largest among the 3 given numbers
Echo “Enter 3 numbers with spaces in between”
Read a b c
1 = $a
if [ $b -gt $l ]
then
l = $b
fi
if [ $c -gt $l ]
then
l = $c
fi
Echo “Largest of $a $b $c is $l”
8) Write a shell program to search for a given number from the list of numbers provided using binary search method
Echo “Enter array limit”
Read limit
Echo “Enter elements”
n = 1
while [ $n -le $limit ]
do
Read num
eval arr$n = $num
n = `expr $n + 1`
done
Echo “Enter key element”
Read key
low = 1
high = $n
found = 0
while [ $found -eq 0 -a $high -gt $low ]
do
mid = `expr ( $low + $high ) / 2`
eval t = $arr$mid
if [ $key -eq $t ]
then
found = 1
elif [ $key -lt $t ]
then
high = `expr $mid – 1`
else
low = `expr $mid + 1`
fi
done
if [ $found -eq 0 ]
then
Echo “Unsuccessful search”
else
Echo “Successful search”
fi
9) Write a shell program to concatenate two strings and find the length of the resultant string
100 Shell Programs In Unix Pdf Download
Echo “Enter first string:”
Read s1
Echo “Enter second string:”
Read s2
s3 = $s1$s2
len = `Echo $s3 | wc -c`
len = `expr $len – 1`
Echo “Concatenated string is $s3 of length $len ”
10) Write a shell program to find the position of substring in given string
Echo “Enter main string:”
Read main
l1 = `Echo $main | wc -c`
l1 = `expr $l1 – 1`
Echo “Enter sub string:”
Read sub
l2 = `Echo $sub | wc -c`
l2 = `expr $l2 – 1`
n = 1
m = 1
pos = 0
while [ $n -le $l1 ]
do
a = `Echo $main | cut -c $n`
b = `Echo $sub | cut -c $m`
if [ $a = $b ]
then
n = `expr $n + 1`
m = `expr $m + 1`
pos = `expr $n – $l2`
r = `expr $m – 1`
if [ $r -eq $l2 ]
then
break
fi
else
pos = 0
m = 1
n = `expr $n + 1`
fi
done
Echo “Position of sub string in main string is $pos”
11) Write a shell program to display the alternate digits in a given 7 digit number starting from the first digit
Echo “Enter a 7 digit number”
Read num
n = 1
while [ $n -le 7 ]
do
a = `Echo $num | cut -c $n`
Echo $a
n = `expr $n + 2`
done
12) Write a shell program to find the gcd for the 2 given numbers
Echo “Enter two numbers with space in between”
Read a b
m = $a
if [ $b -lt $m ]
then
m = $b
fi
while [ $m -ne 0 ]
do
x = `expr $a % $m`
y = `expr $b % $m`
if [ $x -eq 0 -a $y -eq 0 ]
then
Echo “gcd of $a and $b is $m”
break
fi
m = `expr $m – 1`
done
13) Write a shell program to check whether a given string is palindrome or not.
Echo “Enter a string to be entered:”
Read str
Echo
len = `Echo $str | wc -c`
len = `expr $len – 1`
i = 1
j = `expr $len / 2`
while test $i -le $j
do
k = `Echo $str | cut -c $i`
l = `Echo $str | cut -c $len`
if test $k != $l
then
Echo “String is not palindrome”
exit
fi
i = `expr $i + 1`
len = `expr $len – 1`
done
Echo “String is palindrome”
14) Write a shell program to find the sum of the series sum=1+1/2+…+1/n
Echo “Enter a number”
Read n
i = 1
sum = 0
while [ $i -le $n ]
do
sum = `expr $sum + ( 10000 / $i )`
i = `expr $i + 1`
done
Echo “Sum n series is”
i = 1
while [ $i -le 5 ]
do
a = `Echo $sum | cut -c $i`
Echo -e “$ac”
if [ $i -eq 1 ]
then
Echo -e “.c”
fi
i = `expr $i + 1`
done
15) Write a shell script to find the smallest of three numbers
Echo “Enter 3 numbers with spaces in between”
Read a b c
s = $a
if [ $b -lt $s ]
then
s = $b
fi
if [ $c -lt $s ]
then
s = $c
fi
Echo “Smallest of $a $b $c is $s”
16) Write a shell program to add, subtract and multiply the 2 given numbers passed as command line arguments
add = `expr $1 + $2`
sub = `expr $1 – $2`
mul = `expr $1 * $2`
Echo “Addition of $1 and $2 is $add”
Echo “Subtraction of $2 from $1 is $sub”
Echo “Multiplication of $1 and $2 is $mul”
17) Write a shell program to convert all the contents into the uppercase in a particular file
Echo “Enter the filename”
Read filename
Echo “Contents of $filename before converting to uppercase”
Echo —————————————————-
cat $filename
Echo —————————————————-
Echo “Contents of $filename after converting to uppercase”
Echo —————————————————
tr ‘[a-z]’ ‘[A-Z]’ < $filename Echo ————————————————— 18) Write a shell program to count the characters, count the lines and the words in a particular file Echo “Enter the filename” Read file w = `cat $file | wc -w` c = `cat $file | wc -c` l = `grep -c “.” $file` Echo “Number of characters in $file is $c” Echo “Number of words in $file is $w” Echo “Number of lines in $file is $l” 19) Write a shell program to concatenate the contents of 2 files Echo “Enter first filename” Read first Echo “Enter second filename” Read second cat $first > third
cat $second >> third
Echo “After concatenation of contents of entered two files”
Echo —————————————————-
cat third | more
Echo —————————————————-
20) Write a shell program to count number of words, characters, white spaces and special symbols in a given text
Echo “Enter a text”
Read text
w = `Echo $text | wc -w`
w = `expr $w`
c = `Echo $text | wc -c`
c = `expr $c – 1`
s = 0
alpha = 0
j = ` `
n = 1
while [ $n -le $c ]
do
ch = `Echo $text | cut -c $n`
if test $ch = $j
then
s = `expr $s + 1`
fi
case $ch in
a|b|c|d|e|f|g|h|i|j|k|l|m|n|o|p|q|r|s|t|u|v|w|x|y|z) alpha=`expr $alpha + 1`;;
esac
n = `expr $n + 1`
done
special = `expr $c – $s – $alpha`
Echo “Words = $w”
Echo “Characters = $c”
Echo “Spaces = $s”
Echo “Special symbols = $special”
24) Write a shell program to find factorial of given number
Echo “Enter a number”
Read n
fact = 1
i = 1
while [ $i -le $n ]
do
fact = `expr $fact * $i`
i = `expr $i + 1`
done
Echo “Factorial of $n is $fact”
25) Write a shell script to find the average of the numbers entered in command line
n = $#
sum = 0
for i in $*
do
sum = `expr $sum + $i`
done
avg = `expr $sum / $n`
Echo “Average=$avg”
26) Write a shell script to sort the given numbers in descending order using Bubble sort
Echo
i = 1
k = 1
Echo “Enter no. of integers to be sorted”
Read n
Echo “Enter the numbers”
while [ $i -le $n ]
do
Read num
x[$k] = `expr $num`
i = `expr $i + 1`
k = `expr $k + 1`
done
x[$k] = 0
k = 1
Echo “The number you have entered are”
while [ ${x[$k]} -ne 0 ]
do
Echo “${x[$k]}”
k = `expr $k + 1`
done
k = 1
while [ $k -le $n ]
do
j = 1
while [ $j -lt $n ]
do
y = `expr $j + 1`
if [ ${x[$j]} -gt ${x[$y]} ]
then
temp = `expr ${x[$j]}`
x[$j] = `expr ${x[$y]}`
x[$y] = `expr $temp`
fi
j = `expr $j + 1`
done
k = `expr $k + 1`
done
k = 1
Echo “Number in sorted order…”
while [ ${x[$k]} -ne 0 ]
do
Echo “${x[$k]}”
k = `expr $k + 1`
done
27) Write a shell program to find the sum of all the digits in a given 5 digit number
Echo “Enter a 5 digit number”
Read num
sum = 0
while [ $num -ne 0 ]
do
r = `expr $num % 10`
sum = `expr $sum + $r`
num = `expr $num / 10`
done
Echo “sum = $sum”
28) Write a shell script to generate fibonacci series
Echo “how many fibonacci numbers do u want ”
Read limit
a = 0
b = 1
d = 1
Echo “————————————————————-”
Echo -n $a
Echo -n ” ”
while test $d -le $limit
do
c = `expr ${a} + ${b}`
Echo -n $c
Echo -n ” ”
b = $a
a = $c
d = `expr $d + 1`
done
29) Shell Script to check whether given year is leap year or not
Echo -n “Enter the year(yyyy) to find leap year :- ”
Read year
d = `expr $year % 4`
b = `expr $year % 100`
c = `expr $year % 400`
if [ $d -eq 0 -a $b -ne 0 -o $c -eq 0 ]
then
Echo “year is leap year”
else
Echo “not leap year”
fi
30) Shell Script to print alternate digit when a 7 digit number is passed
Echo -n “Enter a 7 digit number:- ”
Read number
len = `echo $number | wc -c`
flag = 1
while test $flag -le $len
do
Echo $number | cut -c$flag
flag = `expr $flag + 2`
done
31) Shell script to find average of number at given command line
total = 0
count = $#
for i #or u can append ( in $*) to get same result.
do
total = `expr $total + $i`
done
avg1 = `expr $total / $count`
avg2 = `expr $total % $count`
avg2 = `expr $avg2 * 100 / $count`
Echo “The Average is :- $avg1.$avg2”
32) Shell Script to reverse a inputted string and show it
Echo -n “enter the string u want to reverse:-”
Read string
len = `Echo -n $string |wc -c`
Echo “no of character is:- $len”
while test $len -gt 0
do
rev = $rev`Echo $string |cut -c $len`
len = `expr $len – 1`
done
Echo “the reverse string is:-$rev ”
33) Shell script to find occurrence of particular digit in inputted number
Echo -n “enter any number:-”
Read number
Echo -n “which digit number do u want to count:-”
Read digit
len = `echo -n $number |wc -c`
Echo “the length of number is:-$len”
count = 0
while test $len -gt 0
do
flag = `Echo -n $number |cut -c $len`
if test $flag -eq $digit
then
count = `expr $count + 1`
fi
len = `expr $len – 1`
done
Echo “|$digit| occurred |$count| times in number ($number)”
34) Shell Script to find whether number given is even or odd
Echo -n “enter any integer number to find even and odd :-”
Read number
rem = `expr $number % 2`
if test $rem -eq 0
then
Echo “number is even”
else
Echo “number is odd”
fi
35) write shell script to generate fibonacci series
#!/bin/bash
#shell script to generate fibonacci series
echo “how many fibonacci numbers do u want ”
read limit
a=0
b=1
d=1
echo “————————————————————-”
echo -n $a
echo -n ” ”
while test $d -le $limit
do
c=`expr ${a} + ${b}`
echo -n $c
echo -n ” ”
b=$a
a=$c
d=`expr $d + 1`
done
36) write shell script to find wheather a particular year is a leap year or not
#!/bin/bash
#shell script to find wheather a particular year is a leap year or not
echo -n “Enter the year(yyyy) to find leap year :- ”
read year
d=`expr $year % 4`
b=`expr $year % 100`
c=`expr $year % 400`
if [ $d -eq 0 -a $b -ne 0 -o $c -eq 0 ]
then
echo “year is leap year”
else
echo “not leap year”
fi
37) write shell script to print alternate digits when a 7 digit number is passed as input
#!/bin/bash
#shell script to print alternate digits when a 7 digit number is passed as input
echo -n “Enter a 7 digit number:- ”
read number
len=`echo $number | wc -c`
flag=1
while test $flag -le $len
do
echo $number | cut -c$flag
flag=`expr $flag + 2`
done
38) write shell script to find average of numbers given at command line
100 Shell Programs In Unix Pdf

#Tue May 28 11:12:41 MUT 2002
total=0
count=$#
for i #or u can append ( in $*) to get same result.
do
total=`expr $total + $i`
done
avg1=`expr $total / $count`
avg2=`expr $total % $count`
avg2=`expr $avg2 * 100 / $count`
echo “The Average is :- $avg1.$avg2”
39) write shell script to find wheather a given word is palindrome or not
#Sun Jun 9 12:06:14 MUT 2002 –By Mukesh
clear
echo -n “enter the name :-”
read name
len=`echo -n $name | wc -c`
echo “Length of the name is :-“$len
while [ $len -gt 0 ]
do
rev=$rev`echo $name | cut -c$len`
len=`expr $len – 1`
done
echo “Reverse of the name is :-“$rev
if [ $name = $rev ]
then echo “It is a palindrome”
else
echo “It is not a palindrome”
fi
40) write shell script to reverse a inputed string and show it.
#!/bin/bash
#shell script to reverse a inputed string and show it.
echo -n “enter the string u want to reverse:-”
read string
len=`echo -n $string |wc -c`
echo “no of character is:- $len”
while test $len -gt 0
do
rev=$rev`echo $string |cut -c $len`
len=`expr $len – 1`
done
echo “the reverse string is:-$rev ”
100 Shell Programs In Unix Pdf Example
41) write shell script to count occurence of a perticular digit in inputted number.
#!/bin/bash
#shell script to count occurence of a perticular digit in inputted number.
echo -n “enter any number:-”
read number
echo -n “which digit number do u want to count:-”
read digit
len=`echo -n $number |wc -c`
echo “the length of number is:-$len”
count=0
while test $len -gt 0
do
flag=`echo -n $number |cut -c $len`
if test $flag -eq $digit
then
count=`expr $count + 1`
fi
len=`expr $len – 1`
done
echo “|$digit| occured |$count| times in number ($number)”
42) write shell script to find even or odd.
100 Shell Programs In Unix Pdf Free
#!/bin/bash
#shell script to find even or odd.
100 Shell Programs In Unix Pdf Online
echo -n “enter any integer number to find even and odd :-”
read number
rem=`expr $number % 2`
if test $rem -eq 0
then
echo “number is even”
else
echo “number is odd”
fi